PiFace Digital 2 - Creare una Web interface in PHP
By: Giovanni De Costanzo | #InternetofThings #IoT #piface #piface digital 2 #pifaceio #Programmazione #Python #raspberry #raspberrypi
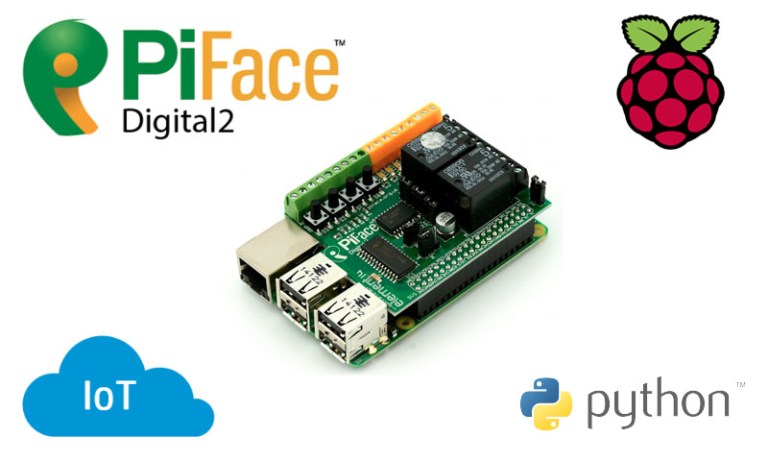
In questo nuovo articolo vedremo come mettere in comunicazione il nostro programma in Python realizzato per la gestione della scheda PiFace con una interfaccia Web, utilizzando PHP.
Per poter mettere in comunicazione il programma scritto in Python con uno script PHP, creeremo delle connessioni socket per lo scambio di messaggi. In particolare, il seguente programma Python fungerà da server, e verrà messo in ascolto sulla porta 9000, in attesa di nuove connessioni client da parte di uno script PHP. Quando viene instaurata una nuova connessione, l’oggetto conn viene passato ad un nuovo thread che, in base al comando ricevuto dal client (nel nostro caso ‘0’, ‘1’ oppure ‘2’), restituisce lo stato della porta di input 0 oppure attiva e disattiva la porta di output 0 (accendendo e spegnendo quindi anche il relativo led).
import socket
import sys
import pifaceio
from thread import *
#---- piaceio read and write functions ------
pfio = pifaceio.PiFace()
def read_pin(pin):
pfio.read()
return pfio.read_pin(pin)
def write_pin(pin, state):
pfio.write_pin(pin, state)
pfio.write()
#---- Socket creation -------
HOST = '' # Symbolic name meaning all available interfaces
PORT = 9000 # Arbitrary non-privileged port
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
print 'Socket created'
try:
s.bind((HOST, PORT))
except socket.error as msg:
print 'Bind failed. Error Code : ' + str(msg[0]) + ' Message ' + msg[1]
sys.exit()
print 'Socket bind complete'
s.listen(10)
print 'Socket now listening'
#---- Function for handling connections. ------
#---- This will be used to create threads -----
def clientthread(conn):
command = conn.recv(1) #Receive 1 Bytes
if command == '0': #Return the state of input port 0
if read_pin(0):
conn.send('1')
else:
conn.send('0')
elif command == '1':
write_pin(0,0)
conn.send('0')
elif command == '2':
write_pin(0,1)
conn.send('0')
#came out of loop
conn.close()
#--- Now waiting for a client connection -----
while 1:
#wait to accept a connection - blocking call
conn, addr = s.accept()
print 'Connected with ' + addr[0] + ':' + str(addr[1])
#start new thread takes 1st argument as a function name to be run
#second is the tuple of arguments to the function.
start_new_thread(clientthread ,(conn,))
s.close()
Di seguito invece è mostrato lo script PHP utilizzato come client il quale, invocato tramite una chiamata AJAX da interfaccia Web, riceve come parametro GET il comando da inviare, si collega al server e invia il comando; resta quindi in attesa di una risposta che sarà poi stampata prima di terminare.
<?php
$host = "localhost";
$port = 9000;
$cmd=$_GET['cmd'] ;
$socket1 = socket_create(AF_INET, SOCK_STREAM,0) or die("Could not create socket\n");
socket_connect ($socket1 , $host,$port ) ;
socket_write($socket1, $cmd, strlen ($cmd)) or die("Could not write output\n");
$response = socket_read($socket1, 1); #read 1 byte
echo $response;
socket_close($socket1) ;
?>